Posted At: Mar 03, 2025 - 613 Views
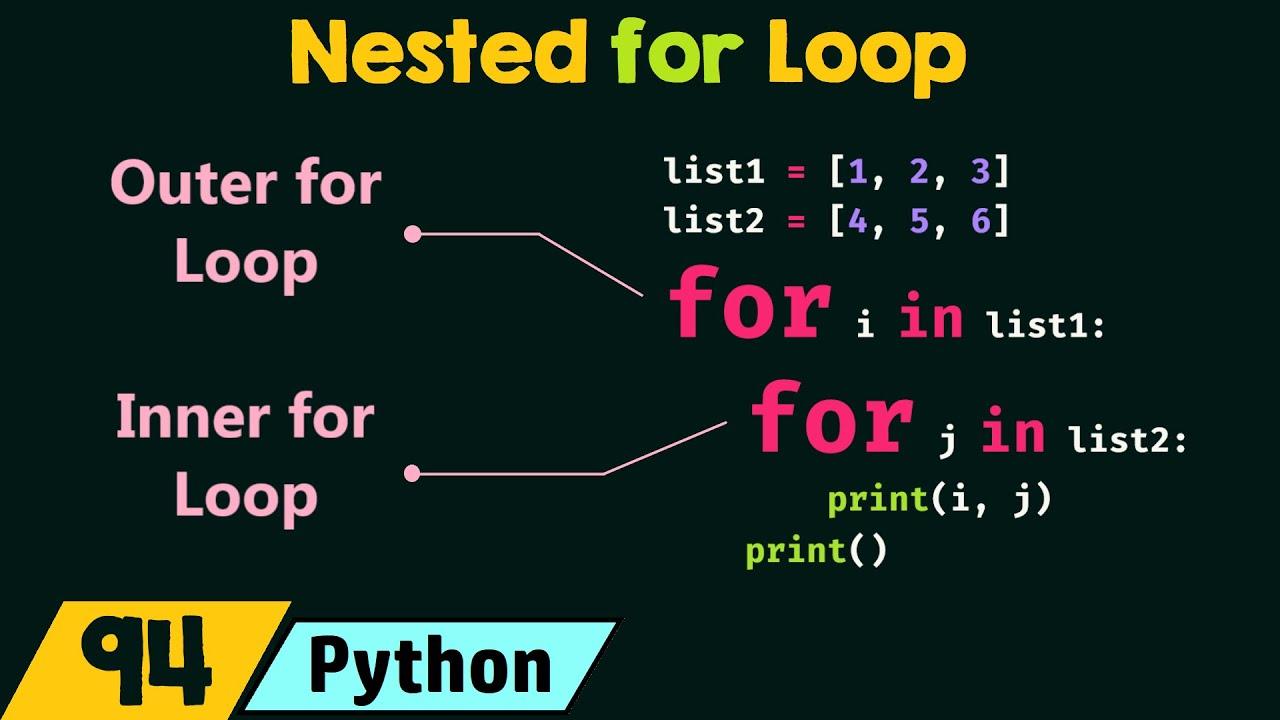
Introduction
Loop structures are a fundamental concept in Python programming, allowing developers to iterate over data structures, automate repetitive tasks, and perform efficient calculations. Among the most widely used loops in Python is the for loop, which provides an elegant and efficient way to traverse lists, tuples, dictionaries, and ranges.
This study explores the structure, advantages, and limitations of Pythonβs for loop, highlighting its role in automation, data analysis, and computational programming.
π Download the Full Guide (PDF): Click Here
1. Understanding the For Loop in Python
π Key Insight: The for loop is a structured way to iterate over a sequence without manually handling counters.
πΉ Basic For Loop Syntax:
for i in range(5):
print("Iteration:", i)
β The loop runs five times, printing values from 0 to 4.
β No need for manual index management, reducing error risks.
πΉ Common Use Cases for For Loops:
β Iterating over lists, tuples, and dictionaries.
β Performing calculations and filtering operations on datasets.
β Automating repetitive data processing tasks.
π‘ Takeaway: Pythonβs for loop provides a simple, readable, and efficient way to handle iterable data structures.
2. Advantages of Using For Loops in Python
π Key Insight: For loops enhance efficiency, readability, and automation in Python programming.
πΉ Benefits of Using For Loops:
β User-Friendly Syntax: Easy for both beginners and experienced developers.
β Error Reduction: No need to manually manage loop counters, reducing bugs.
β Flexibility: Supports lists, tuples, dictionaries, and even ranges, making it versatile for multiple applications.
πΉ Example: Iterating Over a List
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
β The loop iterates through each element in the list, reducing manual effort.
π‘ Takeaway: The for loop is a core component of Python scripting, making iteration simpler and more efficient.
3. Limitations of For Loops & Performance Considerations
π Key Insight: While powerful, for loops have limitations in performance and flexibility.
πΉ Key Limitations of For Loops:
β Requires a predefined sequenceβnot ideal for cases where iterations depend on dynamic conditions.
β Can be slower than while loops for complex calculations or large datasets.
β In performance-critical applications, vectorized operations using NumPy or Pandas may be faster.
πΉ Alternative: While Loop for Dynamic Iteration
count = 0
while count < 5:
print("Iteration:", count)
count += 1
β The while loop runs until a condition is met, making it ideal for non-fixed iteration counts.
π‘ Best Practice: Use for loops for structured iteration and while loops for condition-based repetition.
4. Practical Applications of Loops in Python
β Data Processing: Iterating over datasets to apply calculations and transformations.
β Automation: Repeating tasks like web scraping, API calls, and system monitoring.
β Machine Learning & AI: Handling batch data processing and model training loops.
π‘ Best Practice: Choosing the right loop structure improves performance, readability, and maintainability in Python projects.
Conclusion
For loops are a cornerstone of Python scripting, offering a structured, efficient, and error-free way to handle iteration. While they excel in structured repetition, alternative loop structures like while loops and vectorized operations may be better suited for dynamic or performance-heavy tasks.
π₯ Download Full Guide (PDF): Click Here
Related Python & Programming Resources π
πΉ Python Loops: A Comprehensive Guide
πΉ For Loop vs. While Loop: When to Use Each in Python
πΉ Optimizing Python Code for Performance & Efficiency
π Need expert guidance on Python scripting? π Our professional writers at Highlander Writers can assist with coding tutorials, Python programming guides, and automation projects!
π― Hire a Writer Today!
Leave a comment
Your email address will not be published. Required fields are marked *